meeshoogendoorn
October 18
Simple global state management in Vue using Composables
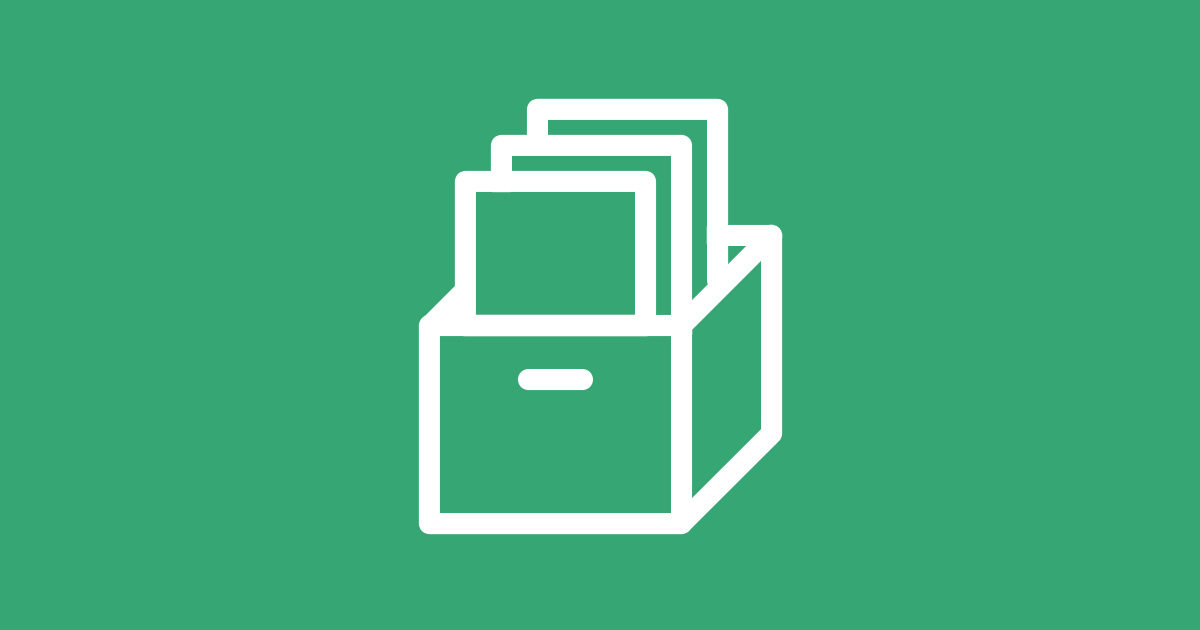
If u want to create a simple global state without using any third party packages like vuex or pinia, we can use the reactives and toRefs props from the Vue Composition api to create our own lightweight state management.
see the following code block:
import { reactive, toRefs } from "@vue/composition-api";
import { loadUsers } from "users.service";
const globalState = reactive({
users: {},
isLoading: {},
});
export default function useUsers() {
const loadUsers = async () => {
globalState.isLoading = true;
try {
globalState.users = await loadUsers();
} catch (e) {
throw Error("[SERVICE][USERS][LOAD] Something went wrong");
} finally {
globalState.isLoading = false;
}
return {
...toRefs(globalState),
loadUsers()
}
}
If u look at the code above u see 2 important props:
reactive & toRefs
the reactive method will create o=a reactive proxy around the object so the object and its values will be observable. the toRefs method will destructure the reactive object and return a plain object to our code.
With above code being so small and simple but we created a performant and lightweight state management in our vue/nuxt application without use of an external state management library lik vuex store or pinia.